Wednesday, October 23, 2019
Sunday, October 20, 2019
phone field in odoo
we can make phone field just as char field but we have to control (validate) the user’s
Create two fields for the phone (the key field and the number field) just like
("phone_num = fields.Char(string="Phon
("phone_key = fields.Char(string="Key")
then create their view to show them just like in the image
<"group colspan="4" col="4>
<";table style="width:40%>
<";tr style="width:100%>
< td style="fonte-size:13px;width:25;>
<b>
Phone
</b>
</td>
<td style="fonte-size:13px;width:15%;">
<field name="phone_key" nolabel="1" placeholder="+249"/>
</td>
<td style="fonte-size:13px;width:60%;">
<field name="phone_num" nolabel="1" placeholder="912312312"/>
</td>
</tr>
</table>
</group>
and finally we have to create the validation function for the phone fields , just like
@api.one
@api.constrains('phone_num','phone_key')
def validate_phone(self):
"""
fuction to validate the input phone number for the user
"""
if len(str(self.phone_key)) != 4:
raise ValidationError(_("Wrong Phone Key"))
if len(str(self.phone_num)) != 9:
raise ValidationError(_("Wrong Phone Number"))
for index in range(0,len(str(self.phone_key))):
if index == 0:
if str(self.phone_key)[index] != '+':
raise ValidationError(_("Wrong Phone Key (It Must Begin With + )"))
if index != 0:
if str(self.phone_key)[index] not in ['0','1','2','3','4','5','6','7','8','9']:
raise ValidationError(_("Wrong Phone Key (It's Conatin Char )"))
for index in range(0,len(str(self.phone_num))):
if str(self.phone_num)[index] not in ['0','1','2','3','4','5','6','7','8','9']:
raise ValidationError(_("Wrong Phone Number (It's Conatin Char )"))
# before creating this function you have to import ValidationError in you model just as
from odoo.exceptions import ValidationError
Thursday, October 3, 2019
period between start date and end date in odoo
We can calculate the
period of time between start date and end date by following the steps
bellow
-
go to the python class and define start_date field which will contain the start date , end_date field which will contain the end date , period_years which will contain the years between the start date and the end date which we will calculate , period_months which will contain the months between the start date and the end date which we will calculate , period_days which will contain the days between the start date and the end date which we will calculate as bellow
start_date = fields.Date(string="Start Date",
required=True)
end_date = fields.Date(string="End Date", required=True)
period_years = fields.Integer(string="Years",
compute="get_period_time")
period_months = fields.Integer(string="Months",
compute="get_period_time")
period_days = fields.Integer(string="Days",
compute="get_period_time")
-
create get_period_time function which will calculate the date between the start date and the end date as
@api.one
def get_period_time(self):
"""
function to calcolate the period of time
when we know the start date and the end date
"""
if self.start_date and self.end_date:
start_date = str(self.start_date)
end_date = str(self.end_date)
start_year_as_int = int(start_date[0]+start_date[1]+start_date[2]+start_date[3])
start_month_as_int = int(start_date[5]+start_date[6])
start_day_as_int = int(start_date[8]+start_date[9])
end_year_as_int = int(end_date[0]+end_date[1]+end_date[2]+end_date[3])
end_month_as_int = int(end_date[5]+end_date[6])
end_day_as_int = int(end_date[8]+end_date[9])
period_years = end_year_as_int-start_year_as_int
period_months = end_month_as_int-start_month_as_int
period_days = end_day_as_int-start_day_as_int
months_list_1 = ['04','06','09','11']
months_list_2 = ['01','03','05','07','08','10','12']
if period_days < 0:
if str(end_month_as_int) == '02':
if end_year_as_int%4 == 0:
period_days = 29+period_days
if end_year_as_int%4 != 0:
period_days = 28+period_days
for index in range(0,4):
if end_month_as_int == int(months_list_1[index]):
period_days = 30+period_days
for index in range(0,7):
if end_month_as_int == int(months_list_2 [index]):
period_days = 31+period_days
period_months = period_months-1
if period_months < 0:
period_months = 12+period_months
period_years = period_years-1
self.period_years = period_years
self.period_months = period_months
self.period_days = period_days
-
design our fields view as below , which will show the fields as in the image
<group colspan="4" col="4">
<field name="start_date"/>
<field name="end_date"/>
<table>
<tr>
<td>
<b style="font-size:13px;">
Period        
</b>
</td>
<td><field name="period_years" nolabel="1"/></td>
<td> Y  -  </td>
<td><field name="period_months" nolabel="1"/></td>
<td> M  -  </td>
<td><field name="period_days" nolabel="1"/></td>
<td> D</td>
</tr>
</table>
</group>
Tuesday, October 1, 2019
Action buttons in odoo
in odoo there are more than one type of button and action button is one of them , so what is an action button ?
An action button is button which be create to call another view , and we can create it by following the steps bellow
- create the button in xml file as
<button name="module_name.view_button_action" icon="fa-check" type="action" string="Graduated Students" />
- create view_graduated_students function as
@api.multi
def view_button_action(self):
return {
'name': _('ModelName'),
'view_type': 'form',
'view_mode': 'tree,form',
'res_model': 'model.model',
'view_id': False,
'type': 'ir.actions.act_window',
'domain': [],
}
'name': _('ModelName'),
'view_type': 'form',
'view_mode': 'tree,form',
'res_model': 'model.model',
'view_id': False,
'type': 'ir.actions.act_window',
'domain': [],
}
cost amount by words in odoo
here we have cost amount money as number and we want to generate cost amount as words by using the number cost amount , and we can do it as bellow
- create the cost amount number field
amount = fields.Float(string="Amount", required=True)
- create the cost amount words field
amount_words = fields.Float(string="Amount In Writting", readonly=True, compute='_onchange_amount')
- create get_amount_in_words function which will convert amount to words and store it in amount_words filed
@api.onchange('amount')
def _onchange_amount(self):
context = self._context or {}
if hasattr(super(ClassName, self), '_onchange_amount'):
super(ClassName, self)._onchange_amount()
if context.get('lang') == 'ar_SY':
units_name = self.currency_id.currency_unit_label
cents_name = self.currency_id.currency_subunit_label
self.amount_words = amount_to_text_ar.amount_to_text(
self.amount, 'ar', units_name, cents_name)
def _onchange_amount(self):
context = self._context or {}
if hasattr(super(ClassName, self), '_onchange_amount'):
super(ClassName, self)._onchange_amount()
if context.get('lang') == 'ar_SY':
units_name = self.currency_id.currency_unit_label
cents_name = self.currency_id.currency_subunit_label
self.amount_words = amount_to_text_ar.amount_to_text(
self.amount, 'ar', units_name, cents_name)
current date in odoo report
we can show the current date in odoo -V11- by multi format as bellow
<span t-esc="context_timestamp(datetime.datetime.now()).strftime('%d/%m/%Y')"/>
<span t-esc="context_timestamp(datetime.datetime.now()).strftime('%Y-%m-%d')"/>
<span t-esc="context_timestamp(datetime.datetime.now()).strftime('
'%Y-%m-%d %H:%M'
')"/>
Subscribe to:
Posts (Atom)
Odoo Invoice Qr code issues
There are two main issues must of us facing with the QR code in Odoo invoice & these issues are 1/ QR code displayed as broken image w...
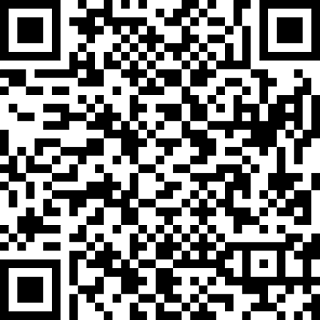
-
ODOO configurations PyPDF2 " ImportError: No module named 'PyPDF2' " this error means that PyPDF2 package is not ...
-
ODOO In this step we will design report for clinic.booking object by wizard so first we have to create wizard in which the use...
-
In this blog we will explain how to send chat message in odoo 16 programmatically #Sending direct chatting message in odoo mail , means sen...